Python
Все задания пожалуйста
Без If and Else,
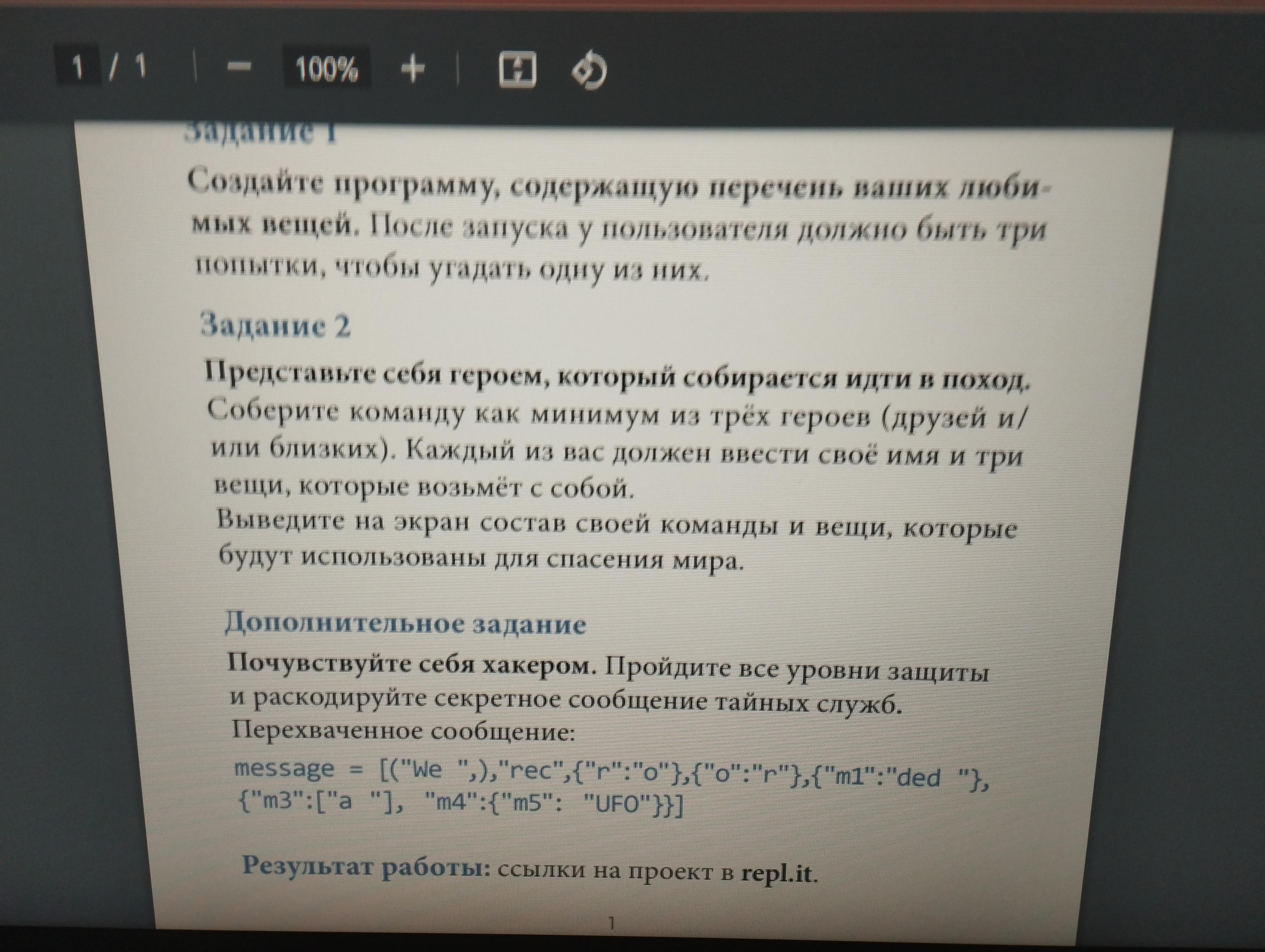
Ответы
import random
def favorite_things_game():
things = ["книги", "компьютерные игры", "музыкальные инструменты"]
thing = random.choice(things)
attempts = 3
print("Добро пожаловать! Попробуйте угадать одну из моих любимых вещей.")
while attempts > 0:
guess = input("Ваша попытка: ")
if guess.lower() == thing.lower():
print("Правильно! Вы угадали.")
break
else:
attempts -= 1
if attempts > 0:
print(f"Неверно. Осталось попыток: {attempts}")
else:
print(f"Извините, вы исчерпали все попытки. Правильный ответ: {thing}")
class Hero:
def __init__(self, name, items):
self.name = name
self.items = items
def create_team():
names = ["Артем", "Ирина", "Максим", "Елена", "Сергей", "Ольга"]
items = ["Меч", "Магический посох", "Лук", "Карта мира", "Зелье лечения", "Трезубец Нептуна"]
return [Hero(random.choice(names), random.sample(items, 3)) for _ in range(3)]
def display_team_info(heroes):
print("Состав команды:")
for hero in heroes:
print(f"{hero.name}: {', '.join(hero.items)}")
def decode_secret_message():
message = [("We",), "rec", {"r":"o"}, {"o":"r"}, {"m1":"ded "}, {"m3":{"a"}}, {"m4":{"m5": "UFO"}}]
decoded_message = "".join([
" ".join(item) if isinstance(item, tuple) else
item if isinstance(item, str) else
"".join([sub_value if isinstance(sub_value, str) else list(sub_value.keys())[0] for sub_key, sub_value in item.items()]) if isinstance(item, dict) and not any(isinstance(sub_key, set) or isinstance(sub_value, set) for sub_key, sub_value in item.items()) else
"" for item in message
])
print(decoded_message)
while True:
task_number = input("Выберите задание (1, 2, 3) или введите 'exit' для завершения: ")
if task_number == "exit":
print("Программа завершена.")
break
elif task_number == "1":
favorite_things_game()
elif task_number == "2":
team = create_team()
display_team_info(team)
elif task_number == "3":
decode_secret_message()
else:
print("Некорректный выбор задания.")