На мові програмування С++
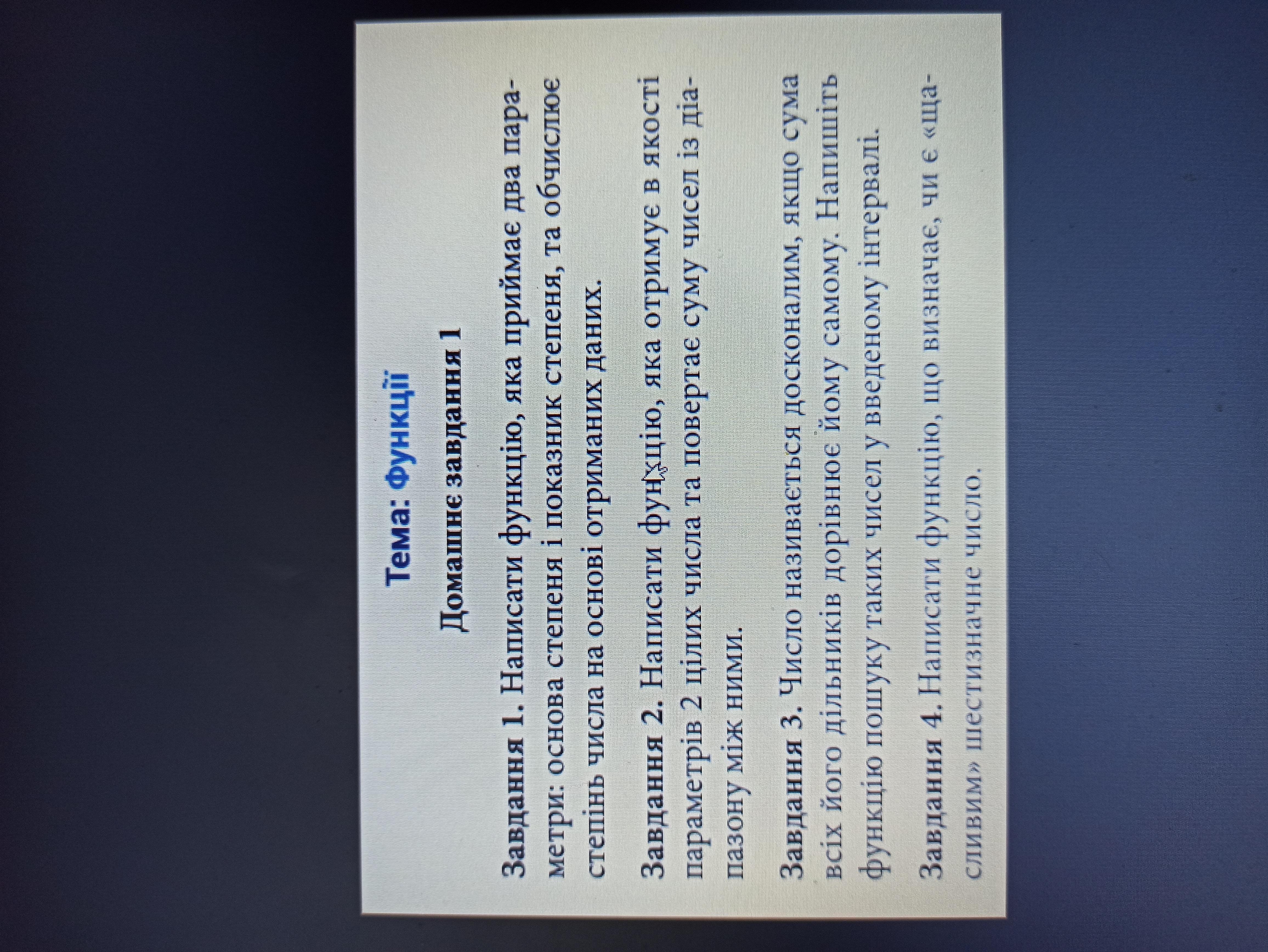
Ответы
Ответ:
Объяснение:
Завдання 1
#include <iostream>
#include <cmath>
using namespace std;
double power(double base, int exponent) {
double result = 1.0;
for (int i = 0; i < abs(exponent); i++) {
result *= base;
}
if (exponent < 0) {
result = 1.0 / result;
}
return result;
}
int main() {
double base;
int exponent;
cout << "Enter base: ";
cin >> base;
cout << "Enter exponent: ";
cin >> exponent;
double result = power(base, exponent);
cout << base << " raised to the power of " << exponent << " is " << result << endl;
return 0;
}
Завдання 2
#include <iostream>
using namespace std;
int sumInRange(int start, int end) {
int sum = 0;
for (int i = start; i <= end; i++) {
sum += i;
}
return sum;
}
int main() {
int start, end;
cout << "Enter the start of the range: ";
cin >> start;
cout << "Enter the end of the range: ";
cin >> end;
int result = sumInRange(start, end);
cout << "The sum of integers in the range [" << start << ", " << end << "] is " << result << endl;
return 0;
}
Завдання 3
#include <iostream>
#include <vector>
std::vector<int> findPerfectNumbers(int start, int end) {
std::vector<int> perfectNumbers;
for (int num = start; num <= end; num++) {
int sum = 0;
for (int i = 1; i <= num / 2; i++) {
if (num % i == 0) {
sum += i;
}
}
if (sum == num) {
perfectNumbers.push_back(num);
}
}
return perfectNumbers;
}
int main() {
int start, end;
std::cout << "Enter the start of the range: ";
std::cin >> start;
std::cout << "Enter the end of the range: ";
std::cin >> end;
std::vector<int> result = findPerfectNumbers(start, end);
if (result.empty()) {
std::cout << "There are no perfect numbers in the range [" << start << ", " << end << "]" << std::endl;
} else {
std::cout << "The perfect numbers in the range [" << start << ", " << end << "] are: ";
for (int num : result) {
std::cout << num << " ";
}
std::cout << std::endl;
}
return 0;
}
Завдання 4
#include <iostream>
using namespace std;
bool isLuckyNumber(int num) {
int firstHalf = num / 1000;
int secondHalf = num % 1000;
int sumFirstHalf = 0;
int sumSecondHalf = 0;
while (firstHalf > 0) {
sumFirstHalf += firstHalf % 10;
firstHalf /= 10;
}
while (secondHalf > 0) {
sumSecondHalf += secondHalf % 10;
secondHalf /= 10;
}
return sumFirstHalf == sumSecondHalf;
}
int main() {
int num;
cout << "Enter a six-digit number: ";
cin >> num;
if (isLuckyNumber(num)) {
cout << num << " is a lucky number!" << endl;
} else {
cout << num << " is not a lucky number." << endl;
}
return 0;
}
Начебто якось так, у 3 я не використовував using namespace std; тому що іноді через це можуть виникати багги