Написать 9 небольших программ на языке Python
Ответы
Sure thing! Here are nine small programs you can write in Python:
1.
print("Привет мир")
2.
name = input("What is your name? ")
print("Hello, " + name + "!")
3.
num = int(input("Please enter a number: "))
square = num * num
print("The square of " + str(num) + " is " + str(square))
4.
n = int(input("Please enter a number: "))
# Initializing the first two numbers of the Fibonacci sequence
a = 0
b = 1
# Printing out the first two numbers
print(a)
print(b)
# Loop to generate and print out the n Fibonacci numbers
for i in range(2, n):
c = a + b
print(c)
a = b
b = c
5.
# Ask the user to enter a list of numbers
numbers = [int(n) for n in input("Please enter a list of numbers separated by spaces: ").split(' ')]
# Calculate the average
total = 0
for num in numbers:
total += num
average = total / len(numbers)
# Print the average
print("The average of the numbers is:", average)
6.
# Ask the user to enter a string
string = input("Please enter a string: ")
# Reverse the string
reversed_string = string[::-1]
# Print the reversed string
print("The reversed string is:", reversed_string)
7.
# Ask the user to enter a number
num = int(input("Please enter a number: "))
# Check if the number is prime
is_prime = True
for i in range(2, num):
if num % i == 0:
is_prime = False
break
# Print the result
if is_prime:
print(str(num) + " is a prime number.")
else:
print(str(num) + " is not a prime number.")
8.
# Ask the user to enter a list of numbers
numbers = [int(n) for n in input("Please enter a list of numbers separated by spaces: ").split(' ')]
# Ask the user if they want to sort the list in ascending or descending order
order = input("Do you want to sort the list in ascending (A) or descending (D) order? ")
# Sort the list
if order == 'A':
numbers.sort()
elif order == 'D':
numbers.sort(reverse=True)
# Print the sorted list
print("The sorted list is:", numbers)
9.
# Ask the user to enter a sentence
sentence = input("Please enter a sentence: ")
# Count the number of words in the sentence
words = sentence.split()
num_words = len(words)
# Print the number of words
print("The number of words in the sentence is:", num_words)
Пояснення:
!!!On the photo is the subject for which the code is written!!!
Sorry for the English, I hope I helped you in some way!
If something does not copy, I have attached below how it should look in the input line
Have a great day!
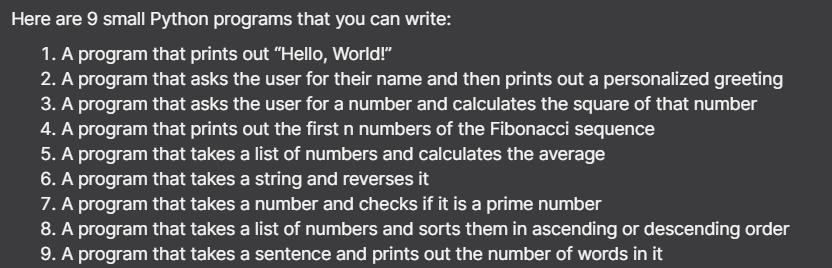