Формат файла - текстовый (храним информацию в виде текста).
Под двоичными потоками подразумевается лучше с FileStream, BinaryStream и тд, но можно StreamWriter и StreamReader.
Под использованием диалогов для ввода данных в файл подразумевается элемент SaveFileDialog(вроде как на скрине 3)
Windows Form C#


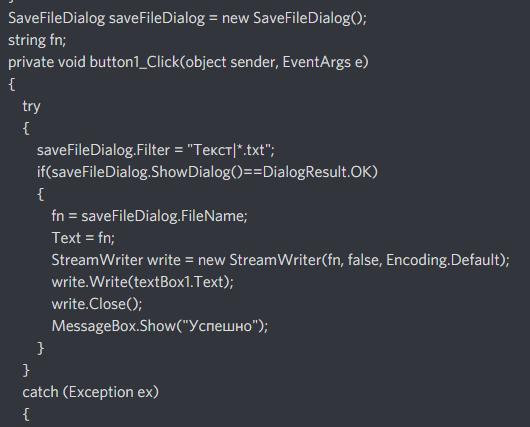
Ответы
Форма:
using System;
using System.Windows.Forms;
namespace WinFormsApp2
{
public partial class Form1 : Form
{
private const int AGE_LIMIT = 20;
public Form1()
{
InitializeComponent();
ActivityComboBox.SelectedIndex = 0;
}
private void ClearFileDataBtn_Click(object sender, EventArgs e)
{
HumansListBox.Items.Clear();
}
private void ClearReadedTextBtn_Click(object sender, EventArgs e)
{
ReadedFileTextBox.Clear();
}
private void WriteToFileBtn_Click(object sender, EventArgs e)
{
var saveDialog = new SaveFileDialog()
{
RestoreDirectory = true,
DefaultExt = "txt"
};
var res = saveDialog.ShowDialog();
if (res != DialogResult.OK || string.IsNullOrEmpty(saveDialog.FileName))
return;
try
{
using (var writer = new StreamWriter(saveDialog.FileName))
{
foreach (Human human in HumansListBox.Items)
{
string data = string.Join(Human.FIELDS_DELIMITER, human.FullName,
human.Birth.ToShortDateString(), human.Activity);
writer.WriteLine(data);
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void GetInfoBtn_Click(object sender, EventArgs e)
{
var openDialog = new OpenFileDialog()
{
RestoreDirectory = true
};
var res = openDialog.ShowDialog();
if (res != DialogResult.OK || string.IsNullOrEmpty(openDialog.FileName))
return;
ReadedFileTextBox.Clear();
try
{
using (var reader = new StreamReader(openDialog.FileName))
{
while (!reader.EndOfStream)
{
string data = reader.ReadLine();
if (string.IsNullOrEmpty(data))
continue;
Human human = Human.Parse(data);
int age = GetAge(human.Birth);
if (age < AGE_LIMIT && human.Activity == Activity.Unemployed)
ReadedFileTextBox.Text += human + Environment.NewLine;
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void AddHumanBtn_Click(object sender, EventArgs e)
{
string data = string.Join(Human.FIELDS_DELIMITER, FullNameTextBox.Text,
BirthTextBox.Text, ActivityComboBox.SelectedItem.ToString());
try
{
Human human = Human.Parse(data);
HumansListBox.Items.Add(human);
}
catch
{
MessageBox.Show("Incorrect data.");
}
}
private void DeleteHumanBtn_Click(object sender, EventArgs e)
{
if (HumansListBox.SelectedIndex != -1)
HumansListBox.Items.RemoveAt(HumansListBox.SelectedIndex);
}
private static int GetAge(DateTime birth)
{
var today = DateTime.Today;
var age = today.Year - birth.Year - 1;
age += (today.Month > birth.Month || today.Month == birth.Month && today.Day >= birth.Day)
? 1
: 0;
return age;
}
}
}
Human:
using System;
using System.Linq;
namespace WinFormsApp2
{
internal enum Activity
{
Working,
Studying,
Unemployed
}
internal struct Human
{
public const string FIELDS_DELIMITER = ",";
public static string HumansDelimiter = Environment.NewLine;
public string FullName { get; }
public DateTime Birth { get; }
public Activity Activity { get; }
public Human(string fullName, DateTime birth, Activity activity)
{
FullName = fullName;
Birth = birth;
Activity = activity;
}
public override string ToString()
{
return $"{FullName} {Birth.ToShortDateString()} {Activity}";
}
public static Human[] ParseMany(string text)
{
return text
.Split(new string[] { HumansDelimiter }, StringSplitOptions.RemoveEmptyEntries)
.Select(t => Parse(t))
.ToArray();
}
public static Human Parse(string text)
{
string[] fields = text.Split(new string[] { FIELDS_DELIMITER },
StringSplitOptions.RemoveEmptyEntries);
string fullName = fields[0];
DateTime birth = DateTime.Parse(fields[1]);
Activity activity = (Activity)Enum.Parse(typeof(Activity), fields[2]);
return new Human(fullName, birth, activity);
}
}
}
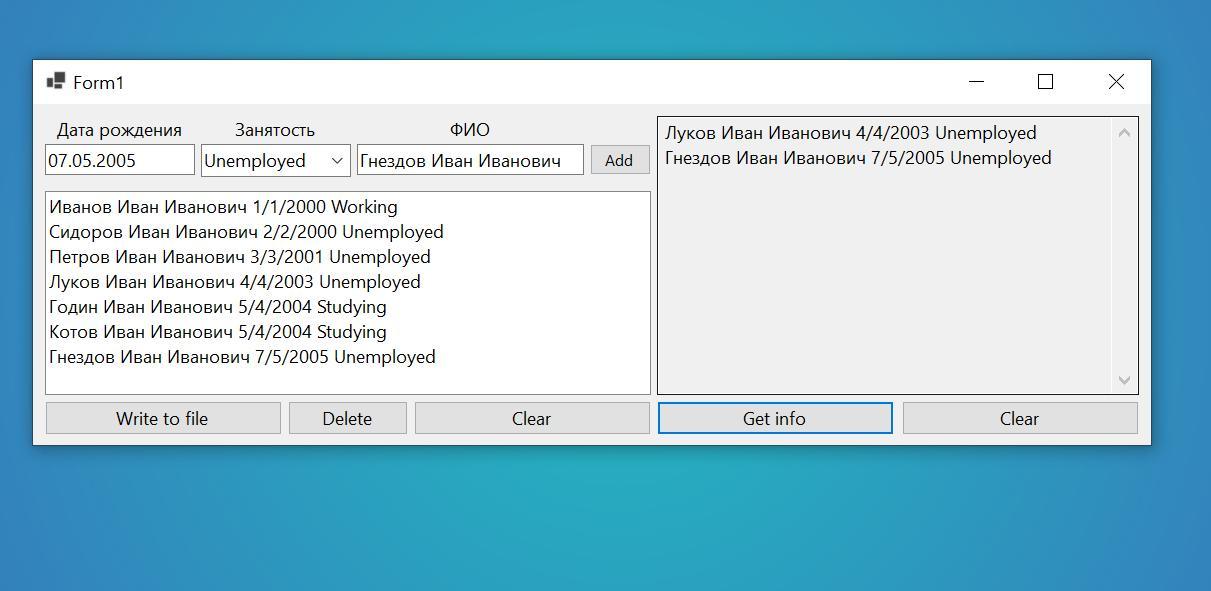
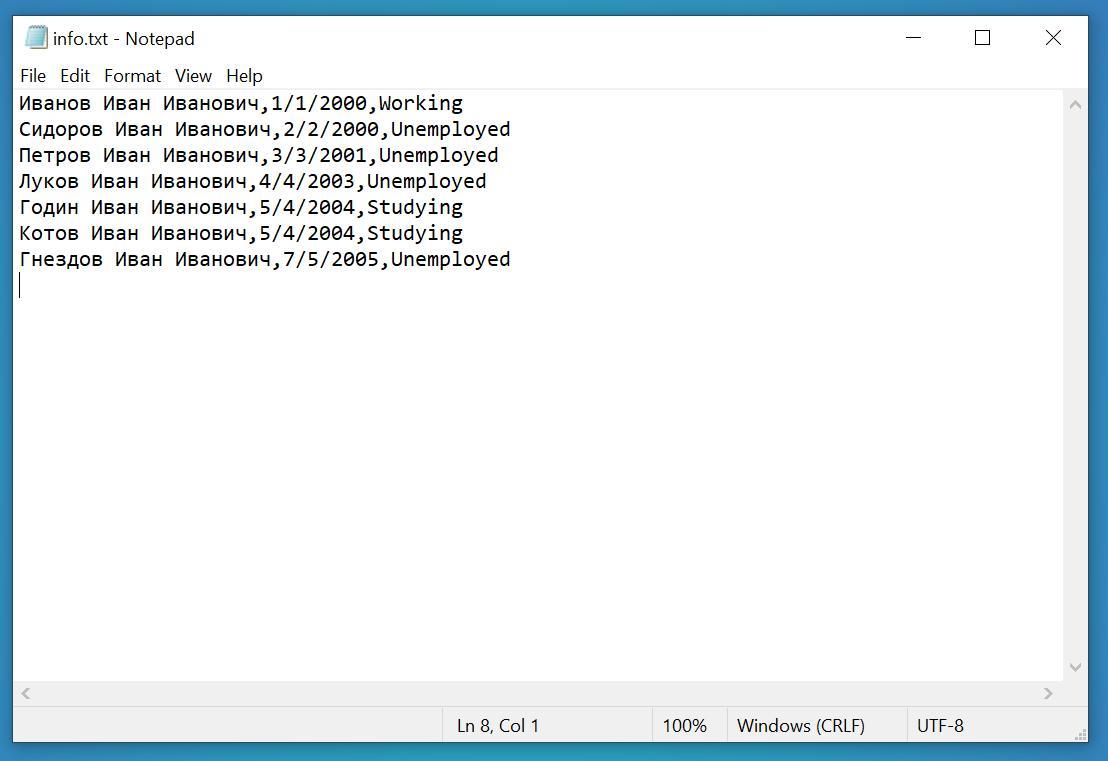